Vue Js Change Image Size with Input Range Slider: In Vue.js, you can use the <input type=”range”> element to create a range slider, which can be used to change the size of an image element. To do this, you need to bind the value of the range slider to the width and height of the image element, which can be done using the v-model directive. The range slider should also have a min and max value that correspond to the minimum and maximum size of the image element. Once this is set up, when the user slides the range slider, the image element will automatically update to the corresponding size. Additionally, you can use a watcher to detect when the size of the image changes and update the range slider accordingly. This allows for a seamless and intuitive experience when changing the size of an image element.In this tutorial we will learn how to chnage image size with input range slider
How to Implement Vue Js Change image size with input range slider
- The code defines a paragraph element that displays the value of the range slider followed by a percent sign. The value is bound to the
rangeSliderValue
property of the Vue instance using the double curly braces syntax. - A div with a class of
slide-container
is created that contains an HTML input element of typerange
. Themin
andmax
attributes define the minimum and maximum values of the slider respectively. Thev-model
directive binds the value of the slider to therangeSliderValue
property of the Vue instance. - An image is added to the HTML, and its source is set to a URL. The
:style
directive dynamically sets the width of the image based on the value of therangeSliderValue
property. The image also has aborderRadius
style applied to it. - The Vue application is created using
Vue.createApp()
. - A
data
object is defined in the Vue instance, which contains a single propertyrangeSliderValue
initialized to 50. - The code will execute and render the HTML with the range slider and the image, which changes width based on the value of the slider.
Vue Js Change image size with input range slider Example
<div id="app">
<p>{{ rangeSliderValue }}%</p>
<div class="slide-container">
<input type="range" min="1" max="100" v-model="rangeSliderValue" class="slider" />
</div>
<div class="img-container">
<img src="https://www.sarkarinaukriexams.com/images/editor/1670265927Capture123243243423.PNG" :style="{
width: `${rangeSliderValue}%`,
borderRadius: '10px',
}" />
</div>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
rangeSliderValue: 50,
}
},
});
app.mount('#app');
</script>
Output of above example
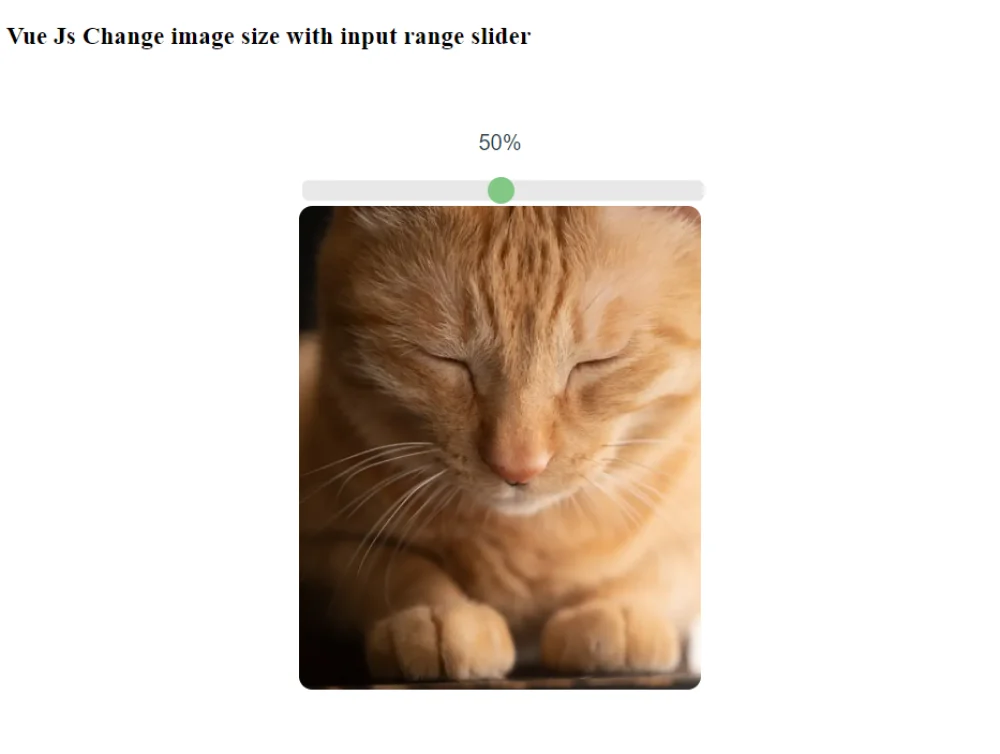